User bio
404 bio not found
Member since Apr 5, 2019
Posts:
Replies:
you have to install it with quote :
pip install "sqlalchemy-iris[intersystems]"
Btw, if you want to avoid conflict between Embedded Python, Community driver and Official one, you can use iris embedded python wrapper :
pip install iris-embedded-python-wrapper
in your case you have to. force-reinstall it :
pip install https://github.com/intersystems-community/intersystems-irispython/releases/download/3.9.2/intersystems_iris-3.9.2-py3-none-any.whl --upgrade --force-reinstall
pip install iris-embedded-python-wrapper --upgrade --force-reinstall
Open Exchange applications:
Certifications & Credly badges:
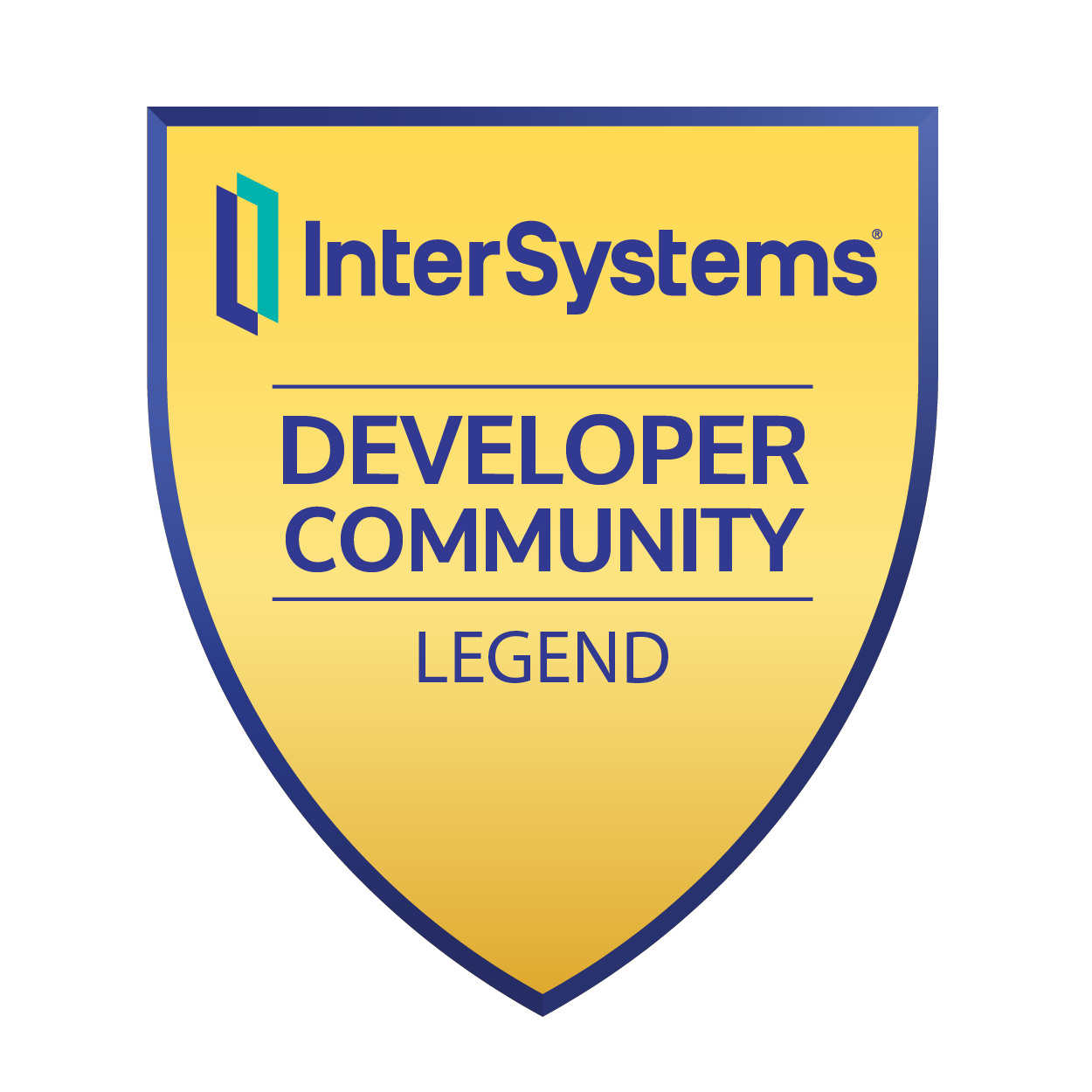
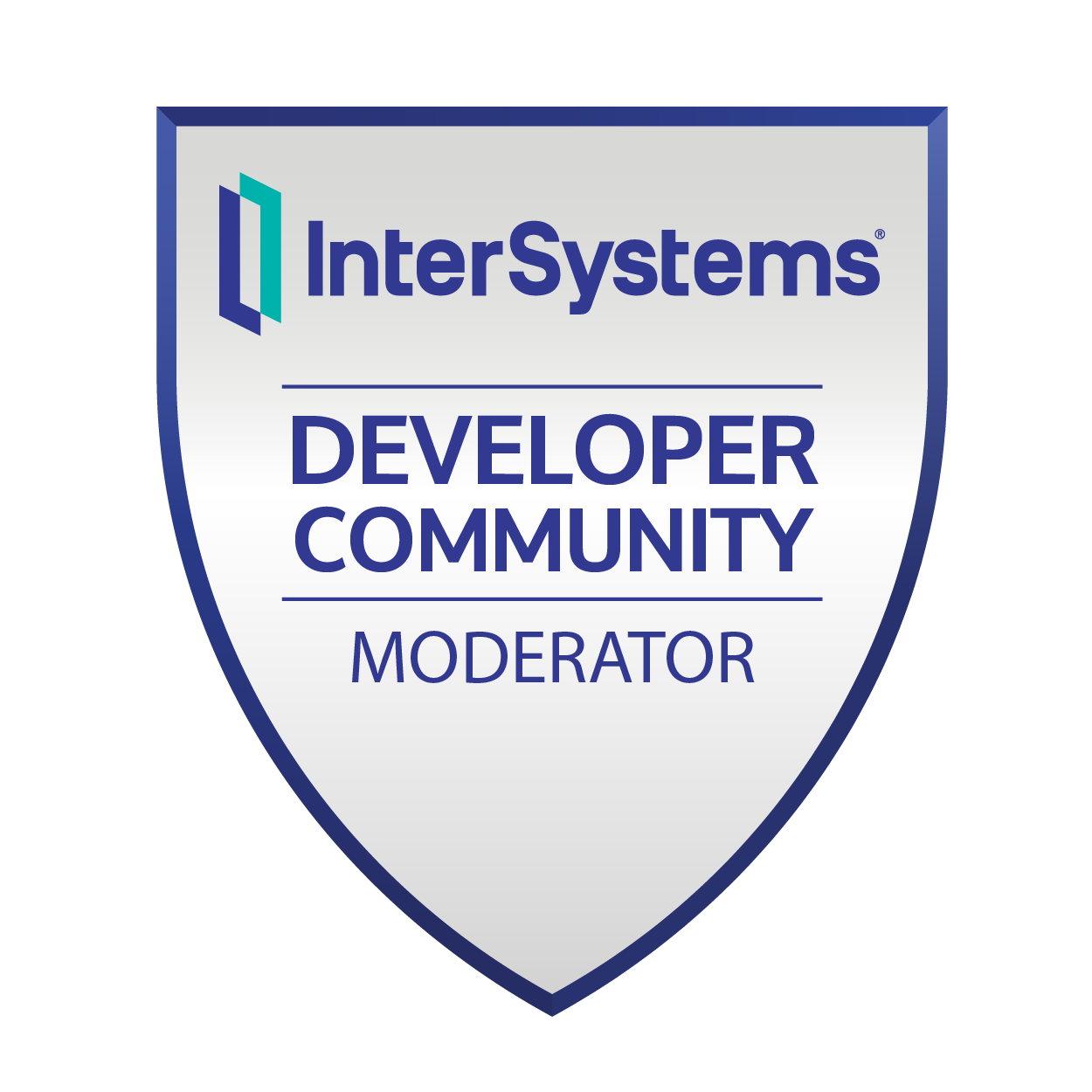
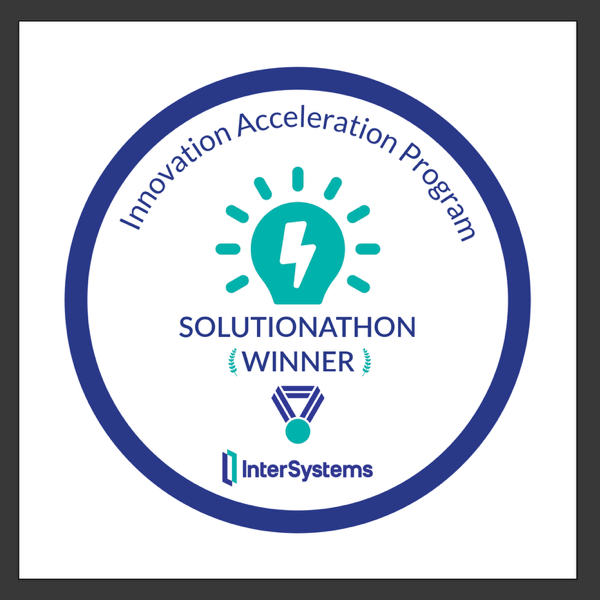
Global Masters badges:
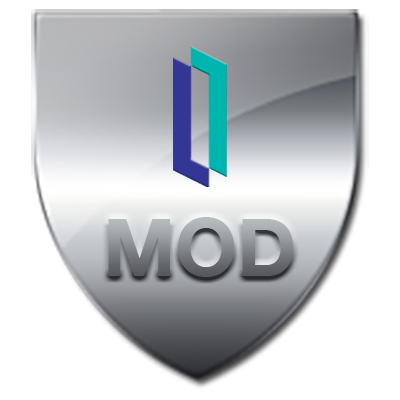
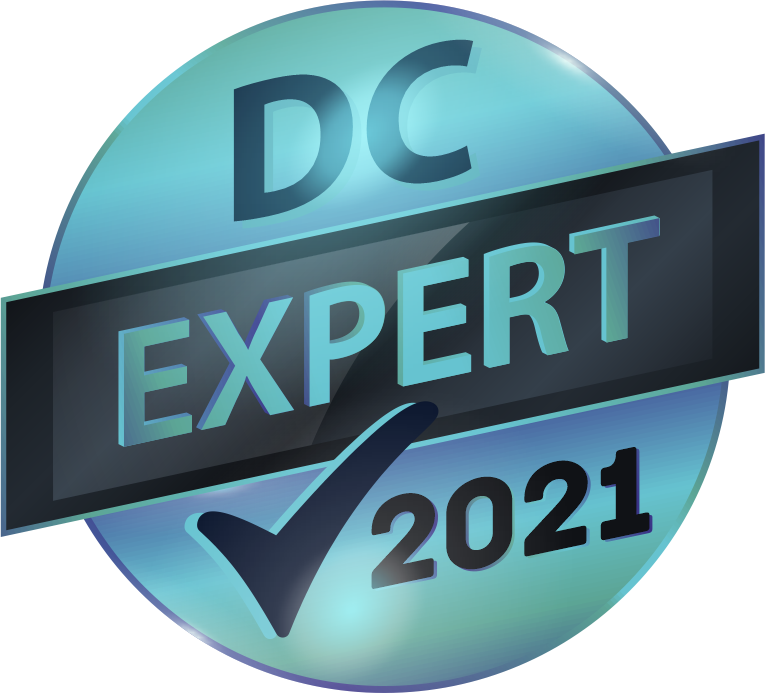
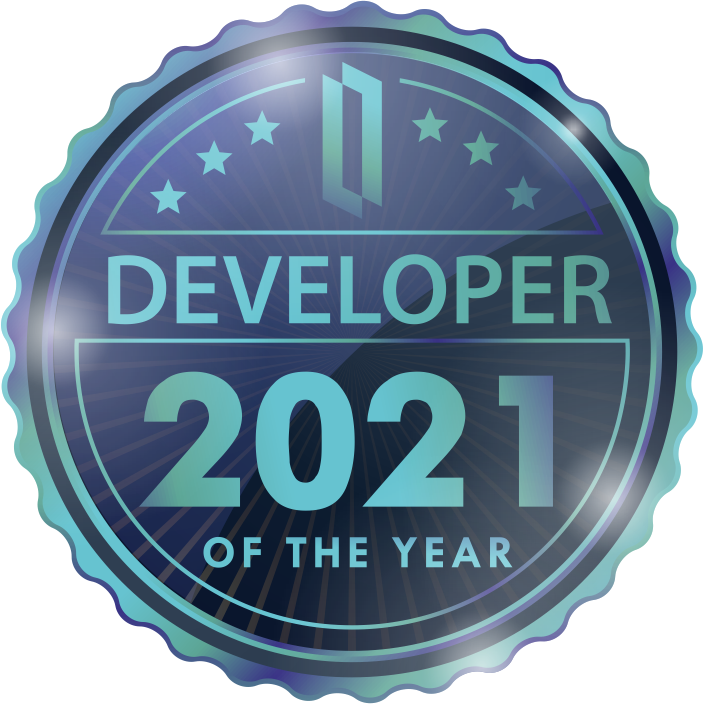
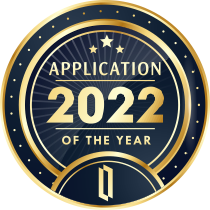
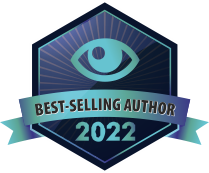
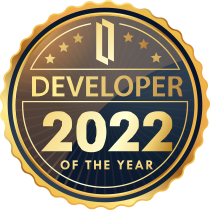
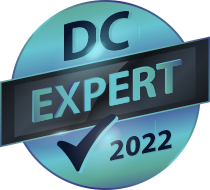
Followers:
Following:
Guillaume has not followed anybody yet.
Hummm, interesting idea, but I think there is some missing context here.
First about objects by themselves. We have Embedded Python that already bridge/bind Python objects to ObjectScript objects. So try to cast a Python object to a %RegisteredObject may not be the optimal way to go. The Embedded Python is already doing that for you.
Second, about Pydantic/ORM. The end goal of this idea is to persist the Pydantic model to a database, right?
There are many ways to do that, I would prefer to stick to the 'pythonic' way of doing things. So, if you want to persist a Pydantic model, I would suggest using SQLAlchemy or SQLModel. They are both great libraries for ORM in Python and have a lot of features that make it easy to work with databases.
Now, if your second goal is to be able to leverage DTL for Python objects, then I would suggest to use an Vdoc approach. You can find a POC here :
https://grongierisc.github.io/interoperability-embedded-python/dtl/
In a nutshell, don't try to bind python way of doing things to ObjectScript. Use the best of both worlds. Use Python for what it is good at and use ObjectScript for what it is good at.