Clear filter
Article
Evgeny Shvarov · Jan 9, 2020
Hi Developers!
Recently we updated the design of Open Exchange and I want to describe how you can find the solutions you are interested in.
Applications Catalog
The first thing you see is the catalog of published applications, tools, and solutions.
The number of applications shows you how many are published or/and fit your query.
You can find the solution you are looking for with the following set of Categories, Products or Industries:
Or you can just search for the tool you are looking for. E.g. let's find applications which help to work with "Python":
Application
The application page shows the Name, version, description, author, publisher (if any), release date, repository, documentation, license, and Download:
Also, it has an active Discuss button if the application has the related post on Developers Community
Every app could have release history:
And issues/tasks open for the application which you can add to a related Github repository:
All the application releases appear on the News page, where you can see the release news and links to the app and author:
You can check all the applications of a particular author. E.g. here are the apps from @Eduard.Lebedyuk:
Applications could be published on behalf of the company. Here are the companies which publish applications on Open Exchange:
And you can check all the applications published by a particular company. E.g. by George James:
This was a quick guide on InterSystems Open Exchange features. In the next article, we'll review how to publish an application on Open Exchange.
Stay tuned!
Announcement
Stefan Wittmann · Aug 5, 2019
I am happy to announce that InterSystems API Manager is now generally available. InterSystems API Manager (IAM) is a new feature of the InterSystems IRIS Data Platform™, enabling you to monitor, control and govern traffic to and from web-based APIs within your IT infrastructure.
The number of API deployments is skyrocketing across industries as more companies build service-oriented application layers. As this number grows, software environments are becoming more distributed, making it critical for API traffic to be properly governed and monitored. InterSystems API Manager provides ease of use by enabling developers to route all traffic through a centralized gateway and forward requests to the appropriate target nodes. Developers using InterSystems API Manager will also be able to:
Monitor all API traffic in a central location allowing users to identify and solve issues;
Control API traffic by throttling throughput, configuring allowable payload sizes, whitelist or blacklist IP addresses, and take endpoints into maintenance mode;
Onboard internal and external developers by providing interactive API documentation through a dedicated and customizable developer portal; and
Secure APIs in one central place.
InterSystems API Manager works with InterSystems IRIS or IRIS for Health 2019.2 and up. (Customers using 2019.2 need a new build, 2019.2.0.109.0, now available from the WRC Software Distribution Site).
In order to take advantage of IAM, partners require an InterSystems IRIS license that includes API Management capabilities. This is available free of charge but InterSystems has to issue a new key to enable the functionality.
The IAM distribution is only available in container format. It can be downloaded from the WRC Software Distribution site.
Documentation is part of the regular InterSystems IRIS documentation: InterSystems API Manager Documentation
The IAM version corresponding to this release is 0.34-1-1.
Additional resources
The official Press Release can be found here: InterSystems IRIS Data Platform 2019.2 introduces API Management capabilities
A short animated overview video: What is InterSystems API Manager
An 8-minute video walking you through some of the key highlights: Introducing InterSystems API Manager
A technical introduction on the developer community is now available: Introducing InterSystems API Manager The IAM distribution is only available in container format.Are there plans for rollouting IAM distribution to other options than container and when they will rollout? Hi Juha! Thanks for the question! What are the issues do you face with API Management with container? Hi Juha,IAM is only available in container format and we have no plans to change that at this point in time. I would like to understand the background of your question. Would you prefer a regular installer and if so, why?Thanks,Stefan Stefan,Great to hear that is is now available. Just something I was waiting for to use in my projects. I updated the announcement adding a link to the technical introduction which I just published. Enjoy! :) Can containerized IAM work with non-containerized InterSystems IRIS? Yes. The first release of InterSystems IRIS that supports IAM and is available on all platforms is 2019.1.1.
Question
Vikram Annadurai · Sep 10, 2019
Hi All,I would like to install Intersystems IRIS studio, So I went this link https://login.intersystems.com/login/SSO.UI.Login.cls?referrer=https%253A//wrc.intersystems.com/wrc/Login.cspBut I am getting the below error. So what will be the next step for me!!! please guide me........ Support should indeed help you out. What exactly are you trying to do? Are you already running an InterSystems IRIS server at your organization and you just want to install Studio to connect to it? Or are you looking to install a local evaluation copy of the data platform as well as the IDE? Note - if you're trying to do the former, you can also download and install Atelier, which is the InterSystems IDE plug-in for Eclipse:https://download.intersystems.com/download/atelier.csp Well, if you installed Eclipse 2019-06, can you still get that plug in? It seems to give me problems getting to the store. Hi Benjamin,No, I am not running an Intersystems IRIS serverI am new to Intersystems IRIS platform, So I need to know Where I can run the IRIS code and Where is the IRIS DB. And how can I find those things.(like cache studio, management portal,and so on...)Your guidance would be helpful for me.Best Vikram No. You need to use Eclipse IDE Photon R with Atelier. Got it; thanks. Vikram ... for now, I suggest that you use the opportunity to try InterSystems IRIS for free hosted in a cloud container:https://www.intersystems.com/try-intersystems-iris-for-free/Keep your eyes open for an announcement during Global Summit about another way that you can get your hands on InterSystems IRIS for evaluation purposes.Thanks!Ben Contact InterSystems Support:
https://www.intersystems.com/support-learning/support/immediate-help/ Instead of Studio, you can try to use VSCode-ObjectScript, which is available without any registration and completely for free.
Announcement
Anastasia Dyubaylo · Apr 5, 2023
Hi Community,
Watch this video to see InterSystems IRIS in action as it is applied to real-world use cases, including business 360 and real-time analytics processing:
⏯ InterSystems IRIS Live Demos @ Global Summit 2022
Presenters:
🗣 @joe.gallant, Manager, Sales Engineering, InterSystems🗣 Wei Wen Lee, Quantitative Development Engineer, Financial Services, InterSystems
Subscribe to InterSystems Developers YouTube to stay up to date!
Announcement
Anastasia Dyubaylo · Feb 28, 2023
Hey Developers,
Enjoy watching the new video on InterSystems Developers YouTube:
⏯ InterSystems Security Development Lifecycle @ Global Summit 2022
InterSystems actively partners with you to meet your security requirements. In this session, you'll learn about InterSystems approach to security and our security practices. This information will help you understand how to receive and use the information critical to developing, deploying, and operating secure applications. Beginner level.
🗣 Presenter: Mark-David McLaughlin, Principal Security Architect, InterSystems
Enjoy it and stay tuned! 👍
Announcement
Anastasia Dyubaylo · Nov 29, 2022
Hi Developers,
Enjoy watching the new video on InterSystems Developers YouTube:
⏯ Using Python with InterSystems IRIS @ Global Summit 2022
Witness all the ways you can use InterSystems IRIS with Python traditional client/server, Embedded Python, and the Python Gateway. Learn when to use each of them.
Presenters🗣 @Robert.Kuszewski, Product Manager, Developer Experience, InterSystems🗣 @Stefan.Wittmann, Product Manager, InterSystems🗣 @Raj.Singh5479, Developer Experience Product Manager, InterSystems
Enjoy it and stay tuned! 👍
Announcement
Anastasia Dyubaylo · Jan 30, 2023
Hey Developers,
It's time to announce the Top Contributors of InterSystems Developer Community for 2022 🎉
We are pleased to reward the most active contributors across all regional DC sites (EN, ES, PT, JP, CN, and FR):
Top Authors
Top Experts
Top Opinion Makers
And a new nomination ... Breakthrough of the Year!
Before we share our best of the best, we'd like to introduce a new exciting badge – Breakthrough of the Year. It is a person who started contributing this year and made the greatest contribution to the development of our Сommunity.
Let's take a closer look at the DC Wall of Fame 2022 and greet everyone with big applause! 👏🏼
Badge's Name
Winners DC
Winners InterSystems
Nomination: Breakthrough of the Year
Given to members who started contributing in 2022 and have the most posts, likes, translations, and views in 2022.
Breakthrough of 2022
@Lucas.Enard2487
@Smythe.Smythee
@Mark.OReilly
–
Nomination: InterSystems Best-selling Author
Given to authors whose articles gathered the maximum amount of views in 2022.
1st place: Gold Best-Selling Author 2022
@Yuri.Gomes
@Toshihiko.Minamoto
2nd place: Silver Best-Selling Author 2022
@Lucas.Enard2487
@Yunfei.Lei
3rd place: Bronze Best-Selling Author 2022
@姚.鑫
@Evgeny.Shvarov
4 - 10th places: Best-Selling Author 2022
@Muhammad.Waseem
@Evgeniy.Potapov
@Robert.Cemper1003
@José.Pereira
@Lorenzo.Scalese
@Irene.Mikhaylova
@Dmitry.Maslennikov
@Guillaume.Rongier7183
@Mihoko.Iijima
@Eduard.Lebedyuk
@Peng.Qiao
@Alberto.Fuentes
@Megumi.Kakechi
@Ricardo.Paiva
Nomination: InterSystems Expert
Given to authors, who got the largest number of accepted answers for 2022.
1st place: Gold Expert 2022
@Robert.Cemper1003
@Eduard.Lebedyuk
2nd place: SilverExpert 2022
@Julius.Kavay
@Yunfei.Lei
3rd place: Bronze Expert 2022
@Vitaliy.Serdtsev2149
@Alexander.Koblov
4 - 10th places: DC Expert 2022
@Dmitry.Maslennikov
@Jeffrey.Drumm
@David.Hockenbroch
@Cristiano.Silva
@John.Murray
@Yaron.Munz8173
@Julian.Matthews7786
@Vic.Sun
@Marc.Mundt
@Timothy.Leavitt
@Guillaume.Rongier7183
@Alex.Woodhead
@Benjamin.Spead
@Evgeny.Shvarov
Nomination: InterSystems Opinion Leader
Given to authors whose posts and answers scored the highest number of likes for 2022.
1st place: Gold Opinion Leader 2022
@Yuri.Gomes
@Angelo.Braga5765
2nd place: Silver Opinion Leader 2022
@Robert.Cemper1003
@Eduard.Lebedyuk
3rd place: Bronze Opinion Leader 2022
@Dmitry.Maslennikov
@Guillaume.Rongier7183
4 - 10th places: DC Opinion Leader 2022
@wang.zhe
@Muhammad.Waseem
@Lorenzo.Scalese
@姚.鑫
@Lucas.Enard2487
@Francisco.López1549
@Julius.Kavay
@Evgeny.Shvarov
@Benjamin.Spead
@Rochael.Ribeiro
@Timothy.Leavitt
@Robert.Kuszewski
@Danusa.Ferreira
@Raj.Singh5479
This list is a good reason to start following some of the great authors of the Developer Community ;)
BIG APPLAUSE TO OUR WINNERS!
Congratulations to all of you and thank you for your great contribution to the InterSystems Developer Community for 2022!
P.S. Please take part in our annual survey and help us become better:
👉🏼 InterSystems Developer Community Annual Survey 2022 👈🏼 Thanks the nominations! About Robert Cemper, he is a diamond expert for me, his contribution and apps are fundamental and fantastic to my personal learning @Yuri.Gomes, @Robert.Cemper1003
¡¡Congratulations!!
And thank you for your contribution and for sharing your knowledge and wisdow!
Congrats to all the contributors! You make the community! Thank you! My heartfelt congratulation to all the contributors! Especially to the French ones 😘 You guys rock! Thanks for all and congratulation to @Robert.Cemper1003 ... our light in our dark doubts I am especially grateful for the very active participation of @Robert.Cemper1003 in our Community :) Thank you, do we get a shiny badge for breakthrough on our profiles? Only just signed up for global masters I think badges are only awarded via Global Masters but I may be mistaken (that is where I saw my badges show up) Hi @Mark.OReilly congratulations on this great achievement!!!We award badges on Global Masters, and after that badges appear on Developer Community profiles as well.I now see your new profile on Global Masters, we will award the badge today, and it will appear within 1 day in your DC profile too :)
Announcement
Jayanth kotla · May 4, 2023
Hi,
This is Jayanth from OAK Technologies.
Hope you are all doing well!!
We have a position for InterSystems IRIS Technology Role for our client if anyone is interested, please drop your resume to jayanth@oaktechinc.com
Job Role: IRIS technology role
Location: Chicago, Illinois (Remote Work)
Contract:1+ Year W2 OR 1099 Contact
Required:
IRIS: inter system creative data technology
FHIR- based healthcare application development
Experience on IHE and any cloud Technology is Preferred.
Thanks, and regards,
Jayanth
OAK TECHNOLOGIES, Inc.
300 E Royal Lane Ste # 130, Irving, TX -75039
C: 972-777-4034
jayanth@oaktechinc.com
Announcement
Anastasia Dyubaylo · Nov 5, 2022
Hey Developers!
Watch this video to get a brief overview of the InterSystems API Manager (IAM) and a deeper dive into new features of IAM 2.3 and 2.8:
⏯ API Management with InterSystems IRIS @ Global Summit 2022
Presenters:🗣 @Stefan.Wittmann, Product Manager, InterSystems 🗣 @Robert.Kuszewski, Product Manager, Developer Experience, InterSystems
Stay tuned for the latest videos on InterSystems Developers YouTube!
Announcement
Anastasia Dyubaylo · Dec 7, 2022
Hi Developers,
Enjoy watching the new video on InterSystems Developers YouTube:
⏯ InterSystems IRIS Cloud SQL @ Global Summit 2022
Learn how to deploy a fully managed, scalable, and secure InterSystems IRIS SQL engine in the cloud in under a minute. We'll show you how to get up and running quickly through the InterSystems Cloud Portal — import your schemas and data and connect easily using InterSystems JDBC, ADO.NET, DB-API, and ODBC client drivers.
Presenters:
🗣 Richard Guidice, Senior Cloud Engineer, InterSystems🗣 @Steven.LeBlanc, Product Specialist, InterSystems
Enjoy it and stay tuned! 👍
Article
Lucas Enard · Nov 27, 2022
Hello everyone, I’m a French student in academical exchange for my fifth year of engineering school and here is my participation in the FHIR for Women's Health contest.
This project is supposed to be seen as the backend of a bigger application. It can be plugged into a Front End app and help you gather information from your patients. It will read your data in local and use a Data Transformation to make it into a FHIR object before sending it to the included local FHIR server.
I wanted to participate because Women's Health is a really important topic that must be discussed more. Using my work you can easily transform any CSV file into a FHIR resource to gather information from your patient on any important subject. It could be used to gather information on pregnant women, then these data ( stored in FHIR in IRIS ) could be analyzed to see if there is any problem or complication. They can also be reviewed by a doctor for example.
See [my GitHub](https://github.com/LucasEnard/Contest-FHIR) and the Open Exchange post linked to this article.
Or see the ReadMe here :
# 1. Contest-FHIR
This is a full python IRIS production that gather information from a csv, use a DataTransformation to make it into a FHIR object and then, save that information to a FHIR server.
This use interop as well as FHIR.
The objective is to help people understand how easy it is to use FHIR.
I didn't have the time to create a csv about women's health, but you can easily imagine this application using a csv on Women's Health.
- [1. Contest-FHIR](#1-contest-fhir)
- [2. Prerequisites](#2-prerequisites)
- [3. Installation](#3-installation)
- [3.1. Installation for development](#31-installation-for-development)
- [3.2. Management Portal and VSCode](#32-management-portal-and-vscode)
- [3.3. Having the folder open inside the container](#33-having-the-folder-open-inside-the-container)
- [4. FHIR server](#4-fhir-server)
- [5. Walkthrough](#5-walkthrough)
- [5.1. Messages and objects](#51-messages-and-objects)
- [5.2. Business Service](#52-business-service)
- [5.3. Business Process](#53-business-process)
- [5.4. Business Operation](#54-business-operation)
- [5.5. Conclusion of the walkthrough](#55-conclusion-of-the-walkthrough)
- [6. Creation of a new DataTransformation](#6-creation-of-a-new-datatransformation)
- [7. What's inside the repo](#7-whats-inside-the-repo)
- [7.1. Dockerfile](#71-dockerfile)
- [7.2. .vscode/settings.json](#72-vscodesettingsjson)
- [7.3. .vscode/launch.json](#73-vscodelaunchjson)
# 2. Prerequisites
Make sure you have [git](https://git-scm.com/book/en/v2/Getting-Started-Installing-Git) and [Docker desktop](https://www.docker.com/products/docker-desktop) installed.
If you work inside the container, as seen in [3.3.](#33-having-the-folder-open-inside-the-container), you don't need to install fhirpy and fhir.resources.
If you are not inside the container, you can use `pip` to install `fhirpy` and `fhir.resources`. Check [fhirpy](https://pypi.org/project/fhirpy/#resource-and-helper-methods) and [fhir.resources](https://pypi.org/project/fhir.resources/) for morte information.
# 3. Installation
## 3.1. Installation for development
Clone/git pull the repo into any local directory e.g. like it is shown below:
```
git clone https://github.com/LucasEnard/fhir-client-python.git
```
Open the terminal in this directory and run:
```
docker-compose up --build
```
This will create the IRIS Framework and the FHIR Server.
Once you are done building enter the Management portal, open the interop tab, open the Production and start it.
Now you will see that the CSV was automatically read from your local files and added to the FHIR server.
This app will periodically read any csv inside the "csv" folder and will send it to the FHIR server, doing the Data Transformation needed.
## 3.2. Management Portal and VSCode
This repository is ready for [VS Code](https://code.visualstudio.com/).
Open the locally-cloned `fhir-client-python` folder in VS Code.
If prompted (bottom right corner), install the recommended extensions.
## 3.3. Having the folder open inside the container
You can be *inside* the container before coding if you wish.
For this, docker must be on before opening VSCode.
Then, inside VSCode, when prompted (in the right bottom corner), reopen the folder inside the container so you will be able to use the python components within it.
The first time you do this it may take several minutes while the container is readied.
If you don't have this option, you can click in the bottom left corner and `press reopen in container` then select `From Dockerfile`
[More information here](https://code.visualstudio.com/docs/remote/containers)

By opening the folder remote you enable VS Code and any terminals you open within it to use the python components within the container.
# 4. FHIR server
To complete this walktrough we will use a fhir server.
This fhir server was already build-in when you cloned and build the container.
The url is `localhost:52773/fhir/r4`
# 5. Walkthrough
Complete walkthrough of the Python IRIS production.
## 5.1. Messages and objects
Objects and messages will hold the information between our services,processes and opeartions.
In the `obj.py` file we have to create a dataclass that match the csv, this will be used to hold the information before doing the DataTransformation.
In our example the organization.csv csv looks like this,
```
active;name;city;country;system;value
true;Name1;city1;country1;phone;050678504
false;Name2;city2;country2;phone;123456789
```
Therefore, the object will look like this,
```python
@dataclass
# > This class represents a simple organization
class BaseOrganization:
active:bool = None
name:str = None
city:str = None
country:str = None
system:str = None
value:str = None
```
In the `msg.py` file, we will have two type of request, the first one hold information of an organization before the DataTransformation and the second one can hold the information of the organization after the DataTransformation.
## 5.2. Business Service
In the `bs.py` file we have the code that allows us to read the csv and for each row of the csv ( so for each organization ), map it into an object we created earlier.
Then, for each of those row ( organization ) we create a request and send it to our process to do the DataTransformation.
```python
# We open the file
with open(self.path + self.filename,encoding="utf-8") as csv:
# We read it and map it using the object BaseOrganization from earlier
reader = DataclassReader(csv, self.fhir_type ,delimiter=";")
# For each of those organization, we can create a request and send it to the process
for row in reader:
msg = OrgaRequest()
msg.organization = row
self.send_request_sync('Python.ProcessCSV',msg)
```
## 5.3. Business Process
In the `bp.py` file we have the DataTransformation, converting a simple python object holding little information to a FHIR R4 object.
Here are the steps to do a DataTransformation using embedded python on our simple organization,
```python
# Creation of the object Organization
organization = Organization()
# Mapping of the information from the request to the Organization object
organization.name = base_orga.name
organization.active = base_orga.active
## Creation of the Address object and mapping of the information
## from the request to the Address object
adress = Address()
adress.country = base_orga.country
adress.city = base_orga.city
### Setting the adress of our organization to the one we created
organization.address = [adress]
## Creation of the ContactPoint object and mapping of the
## information from the request to the ContactPoint object
telecom = ContactPoint()
telecom.value = base_orga.value
telecom.system = base_orga.system
### Setting the telecom of our organization to the one we created
organization.telecom = [telecom]
# Now, our DT is done, we have an object organization that is a
# FHIR R4 object and holds all of our csv information.
```
After that, our mapping is done and our DT is working.
Now, we can send this newly created FHIR R4 resource to our FhirClient that is our operation.
## 5.4. Business Operation
In the `bo.py` file we have the FhirClient, this client creates a connection to a fhir server that will hold the information gathered through the csv.
In this example, we use a local fhir server who doesn't need an api key to connect.
To connect to it we have to use in the on_init function,
```python
if not hasattr(self,'url'):
self.url = 'localhost:52773/fhir/r4'
self.client = SyncFHIRClient(url=self.url)
```
Now, when we receive a message/request, we can, by finding the resource type of the resource we send with our request to the client, create an object readable by the client, and then save it to the fhir server.
```python
# Get the resource type from the request ( here "Organization" )
resource_type = request.resource["resource_type"]
# Create a resource of this type using the request's data
resource = construct_fhir_element(resource_type, request.resource)
# Save the resource to the FHIR server using the client
self.client.resource(resource_type,**json.loads(resource.json())).save()
```
It is to be noted that the fhir client works with any resource from FHIR R4 and to use and change our example, we only need to change the DataTransformation and the object the holds the csv information.
## 5.5. Conclusion of the walkthrough
If you have followed this walkthrough you now know exactly how to read a csv of a represetation of a FHIR R4 resource, use a DataTransformation to make it into a real FHIR R4 object and save it to a server.
# 6. Creation of a new DataTransformation
This repository is ready to code in VSCode with InterSystems plugins.
Open `/src/python` to start coding or using the autocompletion.
**Steps to create a new transformation**
To add a new transformation and use it, the only things you need to do is add your csv named `Patient.csv` ( for example ) in the `src/python/csv` folder.
Then, create an object in `src/python/obj.py` called `BasePatient` that map your csv.
Now create a request in `src/python/msg.py` called `PatientRequest` that has a variable `resource` typed BasePatient.
The final step is the DataTransformation, for this, go to `src/python/bp.py` and add your DT. First add `if isinstance(request, PatientRequest):` and then map your request resource to a fhir.resource Patient.
Now if you go into the management portal and change the setting of the `ServiceCSV` to add `filename=Patient.csv` you can just start the production and see your transformation unfold and you client send the information to the server.
**Detailled steps to create a new transformation**
If you are unsure of what to do or how to do it here is a step by step creation of a new transformation :
Create the file `Patient.csv` n the `src/python/csv` folder and fill it with:
```
family;given;system;value
FamilyName1;GivenName1;phone;555789675
FamilyName2;GivenName2;phone;023020202
```
Our CSV hold a family name, a given name and a phone number for two patients.
In `src/python/obj.py` write :
```python
@dataclass
class BasePatient:
family:str = None
given:str = None
system:str = None
value:str = None
```
In `src/python/msg.py` write:
```python
from obj import BasePatient
@dataclass
class PatientRequest(Message):
resource:BasePatient = None
```
In `src/python/bp.py` write:
```python
from msg import PatientRequest
from fhir.resources.patient import Patient
from fhir.resources.humanname import HumanName
```
In `src/python/bp.py` in the `on_request` function write:
```python
if isinstance(request,PatientRequest):
base_patient = request.resource
patient = Patient()
name = HumanName()
name.family = base_patient.family
name.given = [base_patient.given]
patient.name = [name]
telecom = ContactPoint()
telecom.value = base_patient.value
telecom.system = base_patient.system
patient.telecom = [telecom]
msg = FhirRequest()
msg.resource = patient
self.send_request_sync("Python.FhirClient", msg)
```
Now if you go into the management portal and change the setting of the `ServiceCSV` to add `filename=Patient.csv` you can just stop and restart the production and see your transformation unfold and you client send the information to the server.
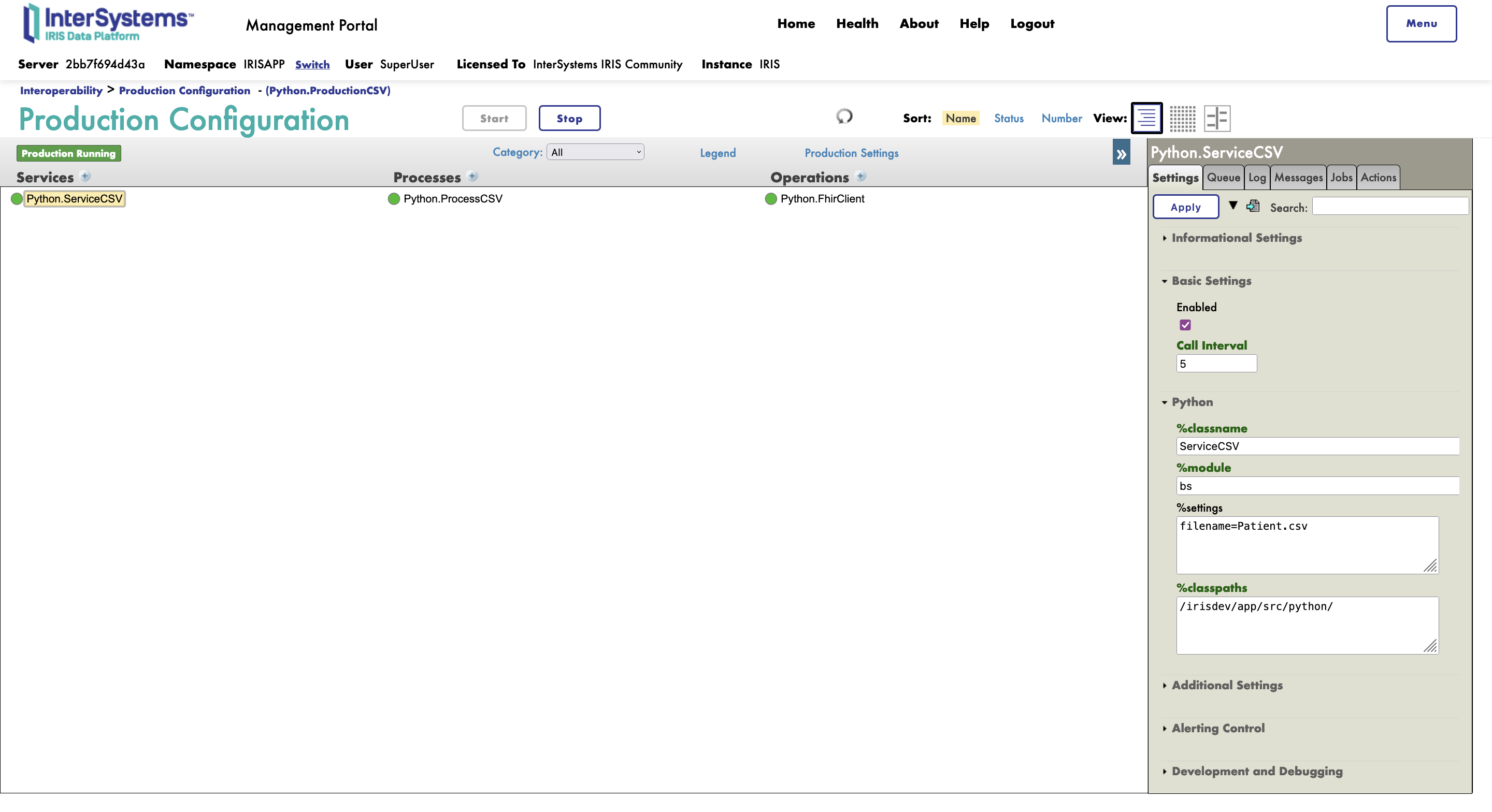
# 7. What's inside the repo
## 7.1. Dockerfile
The simplest dockerfile to start a Python container.
Use `docker-compose up` to build and reopen your file in the container to work inside of it.
## 7.2. .vscode/settings.json
Settings file.
## 7.3. .vscode/launch.json
Config file if you want to debug.
Great example how to convert an CSV file to FHIR.
Thanks for the contribution.
Announcement
Anastasia Dyubaylo · Dec 23, 2022
Hey Developers,
Enjoy watching the new video on InterSystems Developers YouTube:
⏯ Introduction to Analytics with InterSystems IRIS
Get an introduction to the analytics capabilities of InterSystems IRIS data platform and InterSystems IRIS for Health. Learn about the embedded analytics tools and third-party tools supported by InterSystems IRIS and see how you can use these tools for your own analytics needs.
Dive in and stay tuned! 👍
Announcement
Anastasia Dyubaylo · Dec 30, 2022
Hi Developers,
Enjoy watching the new video on InterSystems Developers YouTube:
⏯ InterSystems Cloud Services Overview
See how InterSystems Cloud Services provide targeted, low-barrier solutions to a wide range of issues. Learn how you can simplify your infrastructure, accelerate application development, and reduce the overhead in daily operations and maintenance using these services.
Enjoy watching and stay tuned! 👍 whata great overview of our new Cloud offerings ... well done :)
Announcement
Anastasia Dyubaylo · Jan 6, 2023
Hi Community,
Watch this video to learn about InterSystems FHIR Server, the turnkey FHIR data solution that empowers FHIR application developers to focus on building life-changing healthcare applications:
⏯️ What is InterSystems FHIR Server
Subscribe to InterSystems Developers YouTube to stay tuned for more!