User bio
404 bio not found
Member since Jul 25, 2017
Posts:
Replies:
I can't help with "how to transfer a file to Azure" (I do not use Azure) but before you transfer an IRIS.dat (or an Cache.dat) you should DISMOUNT the corresponding database. Transferring a mounted database is like tire changing in a moving car - it's possible but dangerous.
In the above code just insert a Lock and Unlock, and the problem is solved
//Buid table if it doesn't exist
lock +^LockOutOthers // or any other name you wish
// No timeout! At this point everybody has to wait
// as long as the current job does a table check/update
&SQL(
...
...
}
lock -^LockOutOthers // let the others in
Quit outVisitID
Certifications & Credly badges:
Julius has no Certifications & Credly badges yet.
Global Masters badges:
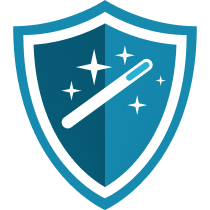
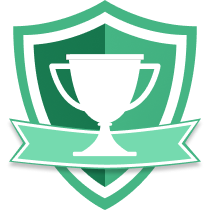
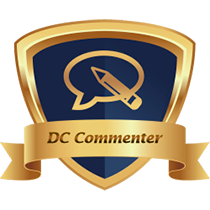
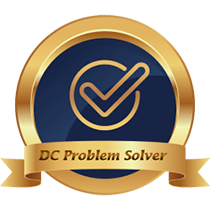

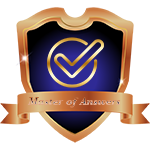
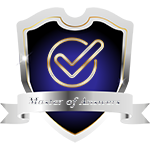
Followers:
Following:
Julius has not followed anybody yet.
The one way is to use %XML.TextReader
ClassMethod Reader(str, pth, ByRef val) { kill val set val=0 // Adjust the method name below to your needs // ParseString(), ParseStream(), ParseFile() if ##class(%XML.TextReader).ParseString(str,.rdr) { while rdr.Read() { if rdr.NodeType="chars",rdr.Path=pth set val($i(val))=rdr.Value } quit 1 } quit 0 } set str="<?xml version=""1.0"" encoding=""UTF-8""?><Input><data><Ids><Id><Type>A</Type><Value>123</Value></Id><Id><Type>B</Type><Value>456</Value></Id></Ids></data></Input>" write ##class(yourClass).Reader(str,"/Input/data/Ids/Id/Value",.val) --> 1 zw val --> val=2 val(1)=123 val(2)=456
The other way is to use %XML.Reader() and correlate to a class which describes your XML structure