User bio
404 bio not found
Member since Nov 11, 2015
Posts:
Replies:
Yes, query Ens.MessageHeader table, maybe joining on a body and then call AbortMessage method here.
But how do you decide on a user? Do you have only one user to assign tasks to?
Open Exchange applications:
Certifications & Credly badges:
Eduard has no Certifications & Credly badges yet.
Global Masters badges:
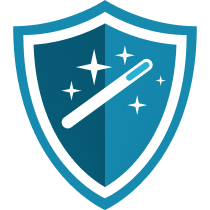
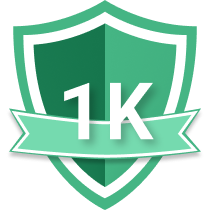
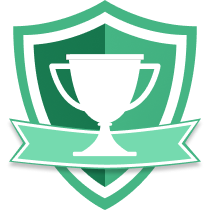
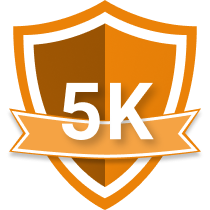
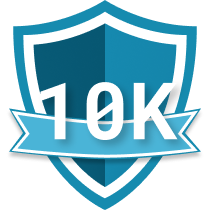
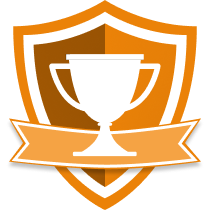
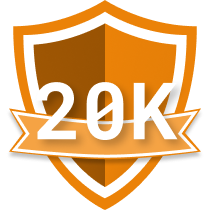
Followers:
Following:
Eduard has not followed anybody yet.
Class methods are recommended for use in all cases.
While classes provide an overhead, this is usually negligible.